diff options
author | Drashna Jaelre <drashna@live.com> | 2018-05-29 08:05:22 -0700 |
---|---|---|
committer | Jack Humbert <jack.humb@gmail.com> | 2018-05-29 11:05:22 -0400 |
commit | 751719e6cb547db753b2f33f06ee6fa61607d544 (patch) | |
tree | 456437ab1d6c051e73ccd2e92d4fb1445d496ca8 | |
parent | 034a25aedf5e0ddba2b05f4b3534c1522330d5cd (diff) |
Add and fix _noeeprom functions to many of the RGB Underglow functions (#3070)0.6.42
* And and fix _noeeprom functions to many of the RGB Underglow functions
* Many functions are unnecessarily calling the eeprom write code. The toggle/enable is command is especially guilty of this, as it writes to EEPROM 3 times. But rgb mode writes twice, every time it's called. And init resets the rgb eeprom range and then writes back to it twice!
* Fixed the rgblight_sethsv_noeeprom to work as expected, by moving a lot of the code to a helper function.
* Added a noeeprom function for mode, enable, disable, and toggle functions. (didn't bother for increase/decrease stuff, and didn't add new keycodes)
* Add to predefined colors list
* Add new functions to manual/docs
* Update RGB Sleep feature to use _noeeprom
Because that's exactly what it should be doing, actually!
-rw-r--r-- | docs/feature_rgblight.md | 35 | ||||
-rw-r--r-- | quantum/rgblight.c | 93 | ||||
-rw-r--r-- | quantum/rgblight.h | 9 | ||||
-rw-r--r-- | quantum/rgblight_list.h | 24 | ||||
-rw-r--r-- | tmk_core/common/avr/suspend.c | 4 |
5 files changed, 137 insertions, 28 deletions
diff --git a/docs/feature_rgblight.md b/docs/feature_rgblight.md index 0f1e649ef8..5369d2fb76 100644 --- a/docs/feature_rgblight.md +++ b/docs/feature_rgblight.md @@ -88,11 +88,36 @@ const uint16_t RGBLED_GRADIENT_RANGES[] PROGMEM = {360, 240, 180, 120, 90}; Look in `rgblights.h` for all available functions, but if you want to control all or some LEDs your goto functions are: ```c -rgblight_disable(); // turn all lights off -rgblight_enable(); // turn lights on, based on their previous state (stored in EEPROM) +// turn all lights off (stored in EEPROM) +rgblight_disable(); +// turn lights on, based on their previous state (stored in EEPROM) +rgblight_enable(); + +// turn all lights off (not stored in EEPROM) +rgblight_disable_noeeprom(); +// turn lights on, based on their previous state (not stored in EEPROM) +rgblight_enable_noeeprom(); + +// where r/g/b is a number from 0..255. Turns all the LEDs to this color (ignores mode, not stored in EEPROM). +rgblight_setrgb(r, g, b); +// HSV color control - h is a value from 0..360 and s/v is a value from 0..255 (stored in EEPROM) +rgblight_sethsv(h, s, v); +// HSV color control - h is a value from 0..360 and s/v is a value from 0..255 (not stored in EEPROM) +rgblight_sethsv_noeeprom(h, s, v); + +// Sets the mode, if rgb animations are enabled (stored in eeprom) +rgblight_mode(x); +// Sets the mode, if rgb animations are enabled (not stored in eeprom) +rgblight_mode_noeeprom(x); +// MODE 1, solid color +// MODE 2-5, breathing +// MODE 6-8, rainbow mood +// MODE 9-14, rainbow swirl +// MODE 15-20, snake +// MODE 21-23, knight +// MODE 24, xmas +// MODE 25-34, static rainbow -rgblight_setrgb(r, g, b); // where r/g/b is a number from 0..255. Turns all the LEDs to this color -rgblight_sethsv(h, s, v); // HSV color control - h is a value from 0..360 and s/v is a value from 0..255 rgblight_setrgb_at(r,g,b, LED); // control a single LED. 0 <= LED < RGBLED_NUM rgblight_sethsv_at(h,s,v, LED); // control a single LED. 0 <= LED < RGBLED_NUM ``` @@ -126,7 +151,7 @@ note: for backwards compatibility, `RGB_SMOD` is an alias for `RGB_MOD`. ## Hardware Modification - +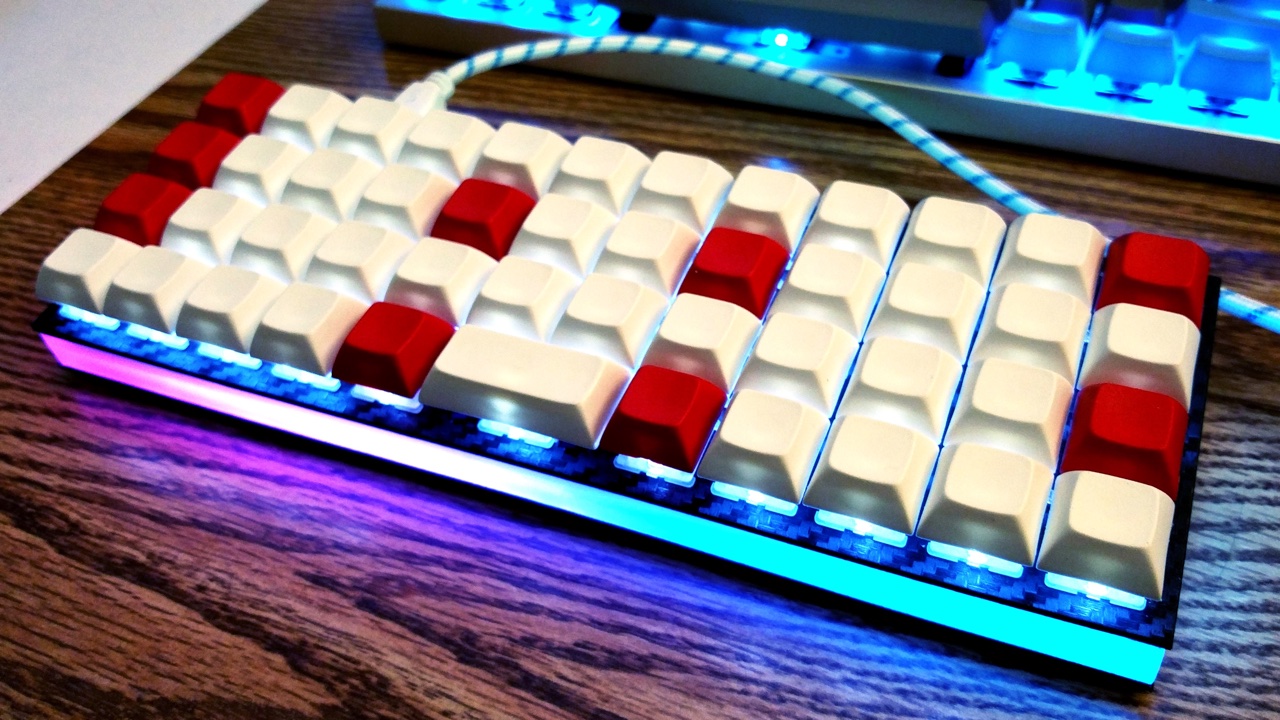 Here is a quick demo on Youtube (with NPKC KC60) (https://www.youtube.com/watch?v=VKrpPAHlisY). diff --git a/quantum/rgblight.c b/quantum/rgblight.c index 75512e97a7..db66e735bb 100644 --- a/quantum/rgblight.c +++ b/quantum/rgblight.c @@ -44,7 +44,6 @@ __attribute__ ((weak)) const uint16_t RGBLED_GRADIENT_RANGES[] PROGMEM = {360, 240, 180, 120, 90}; rgblight_config_t rgblight_config; -rgblight_config_t inmem_config; LED_TYPE led[RGBLED_NUM]; uint8_t rgblight_inited = 0; @@ -161,7 +160,7 @@ void rgblight_init(void) { #endif if (rgblight_config.enable) { - rgblight_mode(rgblight_config.mode); + rgblight_mode_noeeprom(rgblight_config.mode); } } @@ -218,7 +217,7 @@ uint32_t rgblight_get_mode(void) { return rgblight_config.mode; } -void rgblight_mode(uint8_t mode) { +void rgblight_mode_eeprom_helper(uint8_t mode, bool write_to_eeprom) { if (!rgblight_config.enable) { return; } @@ -229,8 +228,12 @@ void rgblight_mode(uint8_t mode) { } else { rgblight_config.mode = mode; } - eeconfig_update_rgblight(rgblight_config.raw); - xprintf("rgblight mode: %u\n", rgblight_config.mode); + if (write_to_eeprom) { + eeconfig_update_rgblight(rgblight_config.raw); + xprintf("rgblight mode [EEPROM]: %u\n", rgblight_config.mode); + } else { + xprintf("rgblight mode [NOEEPROM]: %u\n", rgblight_config.mode); + } if (rgblight_config.mode == 1) { #ifdef RGBLIGHT_ANIMATIONS rgblight_timer_disable(); @@ -254,11 +257,20 @@ void rgblight_mode(uint8_t mode) { rgblight_timer_disable(); #endif } - rgblight_sethsv(rgblight_config.hue, rgblight_config.sat, rgblight_config.val); + rgblight_sethsv_noeeprom(rgblight_config.hue, rgblight_config.sat, rgblight_config.val); +} + +void rgblight_mode(uint8_t mode) { + rgblight_mode_eeprom_helper(mode, true); +} + +void rgblight_mode_noeeprom(uint8_t mode) { + rgblight_mode_eeprom_helper(mode, false); } + void rgblight_toggle(void) { - xprintf("rgblight toggle: rgblight_config.enable = %u\n", !rgblight_config.enable); + xprintf("rgblight toggle [EEPROM]: rgblight_config.enable = %u\n", !rgblight_config.enable); if (rgblight_config.enable) { rgblight_disable(); } @@ -267,17 +279,34 @@ void rgblight_toggle(void) { } } +void rgblight_toggle_noeeprom(void) { + xprintf("rgblight toggle [NOEEPROM]: rgblight_config.enable = %u\n", !rgblight_config.enable); + if (rgblight_config.enable) { + rgblight_disable_noeeprom(); + } + else { + rgblight_enable_noeeprom(); + } +} + void rgblight_enable(void) { rgblight_config.enable = 1; - eeconfig_update_rgblight(rgblight_config.raw); - xprintf("rgblight enable: rgblight_config.enable = %u\n", rgblight_config.enable); + // No need to update EEPROM here. rgblight_mode() will do that, actually + //eeconfig_update_rgblight(rgblight_config.raw); + xprintf("rgblight enable [EEPROM]: rgblight_config.enable = %u\n", rgblight_config.enable); rgblight_mode(rgblight_config.mode); } +void rgblight_enable_noeeprom(void) { + rgblight_config.enable = 1; + xprintf("rgblight enable [NOEEPROM]: rgblight_config.enable = %u\n", rgblight_config.enable); + rgblight_mode_noeeprom(rgblight_config.mode); +} + void rgblight_disable(void) { rgblight_config.enable = 0; eeconfig_update_rgblight(rgblight_config.raw); - xprintf("rgblight disable: rgblight_config.enable = %u\n", rgblight_config.enable); + xprintf("rgblight disable [EEPROM]: rgblight_config.enable = %u\n", rgblight_config.enable); #ifdef RGBLIGHT_ANIMATIONS rgblight_timer_disable(); #endif @@ -285,6 +314,17 @@ void rgblight_disable(void) { rgblight_set(); } +void rgblight_disable_noeeprom(void) { + rgblight_config.enable = 0; + xprintf("rgblight disable [noEEPROM]: rgblight_config.enable = %u\n", rgblight_config.enable); + #ifdef RGBLIGHT_ANIMATIONS + rgblight_timer_disable(); + #endif + _delay_ms(50); + rgblight_set(); +} + + // Deals with the messy details of incrementing an integer uint8_t increment( uint8_t value, uint8_t step, uint8_t min, uint8_t max ) { int16_t new_value = value; @@ -358,23 +398,22 @@ void rgblight_decrease_speed(void) { eeconfig_update_rgblight(rgblight_config.raw);//EECONFIG needs to be increased to support this } -void rgblight_sethsv_noeeprom(uint16_t hue, uint8_t sat, uint8_t val) { - inmem_config.raw = rgblight_config.raw; +void rgblight_sethsv_noeeprom_old(uint16_t hue, uint8_t sat, uint8_t val) { if (rgblight_config.enable) { LED_TYPE tmp_led; sethsv(hue, sat, val, &tmp_led); - inmem_config.hue = hue; - inmem_config.sat = sat; - inmem_config.val = val; // dprintf("rgblight set hue [MEMORY]: %u,%u,%u\n", inmem_config.hue, inmem_config.sat, inmem_config.val); rgblight_setrgb(tmp_led.r, tmp_led.g, tmp_led.b); } } -void rgblight_sethsv(uint16_t hue, uint8_t sat, uint8_t val) { + +void rgblight_sethsv_eeprom_helper(uint16_t hue, uint8_t sat, uint8_t val, bool write_to_eeprom) { if (rgblight_config.enable) { if (rgblight_config.mode == 1) { // same static color - rgblight_sethsv_noeeprom(hue, sat, val); + LED_TYPE tmp_led; + sethsv(hue, sat, val, &tmp_led); + rgblight_setrgb(tmp_led.r, tmp_led.g, tmp_led.b); } else { // all LEDs in same color if (rgblight_config.mode >= 2 && rgblight_config.mode <= 5) { @@ -399,11 +438,23 @@ void rgblight_sethsv(uint16_t hue, uint8_t sat, uint8_t val) { rgblight_config.hue = hue; rgblight_config.sat = sat; rgblight_config.val = val; - eeconfig_update_rgblight(rgblight_config.raw); - xprintf("rgblight set hsv [EEPROM]: %u,%u,%u\n", rgblight_config.hue, rgblight_config.sat, rgblight_config.val); + if (write_to_eeprom) { + eeconfig_update_rgblight(rgblight_config.raw); + xprintf("rgblight set hsv [EEPROM]: %u,%u,%u\n", rgblight_config.hue, rgblight_config.sat, rgblight_config.val); + } else { + xprintf("rgblight set hsv [NOEEPROM]: %u,%u,%u\n", rgblight_config.hue, rgblight_config.sat, rgblight_config.val); + } } } +void rgblight_sethsv(uint16_t hue, uint8_t sat, uint8_t val) { + rgblight_sethsv_eeprom_helper(hue, sat, val, true); +} + +void rgblight_sethsv_noeeprom(uint16_t hue, uint8_t sat, uint8_t val) { + rgblight_sethsv_eeprom_helper(hue, sat, val, false); +} + uint16_t rgblight_get_hue(void) { return rgblight_config.hue; } @@ -546,7 +597,7 @@ void rgblight_effect_breathing(uint8_t interval) { // http://sean.voisen.org/blog/2011/10/breathing-led-with-arduino/ val = (exp(sin((pos/255.0)*M_PI)) - RGBLIGHT_EFFECT_BREATHE_CENTER/M_E)*(RGBLIGHT_EFFECT_BREATHE_MAX/(M_E-1/M_E)); - rgblight_sethsv_noeeprom(rgblight_config.hue, rgblight_config.sat, val); + rgblight_sethsv_noeeprom_old(rgblight_config.hue, rgblight_config.sat, val); pos = (pos + 1) % 256; } void rgblight_effect_rainbow_mood(uint8_t interval) { @@ -557,7 +608,7 @@ void rgblight_effect_rainbow_mood(uint8_t interval) { return; } last_timer = timer_read(); - rgblight_sethsv_noeeprom(current_hue, rgblight_config.sat, rgblight_config.val); + rgblight_sethsv_noeeprom_old(current_hue, rgblight_config.sat, rgblight_config.val); current_hue = (current_hue + 1) % 360; } void rgblight_effect_rainbow_swirl(uint8_t interval) { diff --git a/quantum/rgblight.h b/quantum/rgblight.h index a6593af98b..569424506b 100644 --- a/quantum/rgblight.h +++ b/quantum/rgblight.h @@ -134,7 +134,16 @@ void rgb_matrix_decrease(void); void sethsv(uint16_t hue, uint8_t sat, uint8_t val, LED_TYPE *led1); void setrgb(uint8_t r, uint8_t g, uint8_t b, LED_TYPE *led1); + void rgblight_sethsv_noeeprom(uint16_t hue, uint8_t sat, uint8_t val); +void rgblight_mode_noeeprom(uint8_t mode); +void rgblight_toggle_noeeprom(void); +void rgblight_enable_noeeprom(void); +void rgblight_disable_noeeprom(void); + +void rgblight_sethsv_eeprom_helper(uint16_t hue, uint8_t sat, uint8_t val, bool write_to_eeprom); +void rgblight_mode_eeprom_helper(uint8_t mode, bool write_to_eeprom); + #define EZ_RGB(val) rgblight_show_solid_color((val >> 16) & 0xFF, (val >> 8) & 0xFF, val & 0xFF) void rgblight_show_solid_color(uint8_t r, uint8_t g, uint8_t b); diff --git a/quantum/rgblight_list.h b/quantum/rgblight_list.h index 29b2802263..407fd8e9d3 100644 --- a/quantum/rgblight_list.h +++ b/quantum/rgblight_list.h @@ -77,6 +77,30 @@ #define rgblight_sethsv_pink() rgblight_sethsv (330, 128, 255) /* SET HSV List */ +/* If you're doing layer indication, this is best, as it won't */ +/* write to the eeprom, since it's limited (very high value). */ +/* If you want to use modes with this (since you can), then you */ +/* want to use rgblight_mode_noeeprom(x) instead. */ +#define rgblight_sethsv_noeeprom_white() rgblight_sethsv_noeeprom ( 0, 0, 255) +#define rgblight_sethsv_noeeprom_red() rgblight_sethsv_noeeprom ( 0, 255, 255) +#define rgblight_sethsv_noeeprom_coral() rgblight_sethsv_noeeprom ( 16, 176, 255) +#define rgblight_sethsv_noeeprom_orange() rgblight_sethsv_noeeprom ( 39, 255, 255) +#define rgblight_sethsv_noeeprom_goldenrod() rgblight_sethsv_noeeprom ( 43, 218, 218) +#define rgblight_sethsv_noeeprom_gold() rgblight_sethsv_noeeprom ( 51, 255, 255) +#define rgblight_sethsv_noeeprom_yellow() rgblight_sethsv_noeeprom ( 60, 255, 255) +#define rgblight_sethsv_noeeprom_chartreuse() rgblight_sethsv_noeeprom ( 90, 255, 255) +#define rgblight_sethsv_noeeprom_green() rgblight_sethsv_noeeprom (120, 255, 255) +#define rgblight_sethsv_noeeprom_springgreen() rgblight_sethsv_noeeprom (150, 255, 255) +#define rgblight_sethsv_noeeprom_turquoise() rgblight_sethsv_noeeprom (174, 90, 112) +#define rgblight_sethsv_noeeprom_teal() rgblight_sethsv_noeeprom (180, 255, 128) +#define rgblight_sethsv_noeeprom_cyan() rgblight_sethsv_noeeprom (180, 255, 255) +#define rgblight_sethsv_noeeprom_azure() rgblight_sethsv_noeeprom (186, 102, 255) +#define rgblight_sethsv_noeeprom_blue() rgblight_sethsv_noeeprom (240, 255, 255) +#define rgblight_sethsv_noeeprom_purple() rgblight_sethsv_noeeprom (270, 255, 255) +#define rgblight_sethsv_noeeprom_magenta() rgblight_sethsv_noeeprom (300, 255, 255) +#define rgblight_sethsv_noeeprom_pink() rgblight_sethsv_noeeprom (330, 128, 255) + +/* SET HSV List */ #define rgblight_sethsv_white_at(at) rgblight_sethsv_at ( 0, 0, 255, at) #define rgblight_sethsv_red_at(at) rgblight_sethsv_at ( 0, 255, 255, at) #define rgblight_sethsv_coral_at(at) rgblight_sethsv_at ( 16, 176, 255, at) diff --git a/tmk_core/common/avr/suspend.c b/tmk_core/common/avr/suspend.c index 81e4266410..3aa3d12474 100644 --- a/tmk_core/common/avr/suspend.c +++ b/tmk_core/common/avr/suspend.c @@ -101,7 +101,7 @@ static void power_down(uint8_t wdto) #ifdef RGBLIGHT_ANIMATIONS rgblight_timer_disable(); #endif - rgblight_disable(); + rgblight_disable_noeeprom(); #endif // TODO: more power saving // See PicoPower application note @@ -157,7 +157,7 @@ void suspend_wakeup_init(void) #endif led_set(host_keyboard_leds()); #ifdef RGBLIGHT_SLEEP - rgblight_enable(); + rgblight_enable_noeeprom(); #ifdef RGBLIGHT_ANIMATIONS rgblight_timer_enable(); #endif |